Sai Display Board
Saturday, May 17, 2014
How to Display any Character on a 7 Segment LED Display
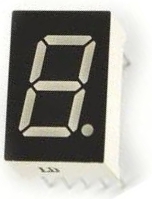
In this project, we will show how you can display any character that is capable
of being displayed on a 7 segment LED display.
All numeral characters can be displayed on a 7 segment display. In fact, in
the article,
How to Drive a 7 Segment LED Display with an Arduino, we programmed the circuit so that it displayed
numerals 0-9 a second apart from each other.
In this project, we simply go over (again) how you can display any character
(which can be displayed) on a 7 segment LED display.
To do this, let us first go over the internal makeup of a 7 segment LED display.
The display is a device that is made up of 8 individual LEDs, including the decimal point
at the bottom. Depending on which LED is lit decides what type of character will be shown.
As an example, look at the numbers shown below.
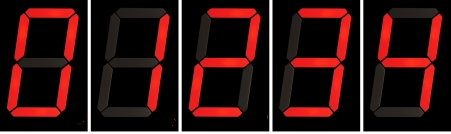
You can easily see which LEDs are lit decides the different numerals shown.
Using this device, we can display all numerals and many alphabetical
characters and many
more types of symbols. The 7 segment LED is really a versatile display device.
For this project, we will show how to create all the alphabetical characters
which can be shown at a 7 segment LED dsiplay. This includes alphabet characters, A, b, C, c, d,
E, F, H, h, L, l, O, o, P, S. These are pretty much the only characters of the alphabet which can be
produced.
The output is produced by turning on combinations of segments that represent
these various alphabetical characters.
Components Needed
- Common Cathode 7 Segment LED Display
- Arduino
- 8 270Ω Resistors
- Jumper Wires
The 270Ω resistors attach to the 8 digital output pins
connected to the 8 segments of the LED display. They limit current going
to the individual LEDs, so that they don't
get burnt out from too much power.
To understand how this program works, let's first look at the schematic makeup of a 7 segment LED
display.
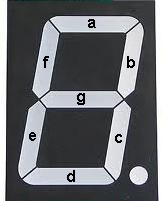
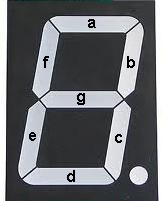
The LED display, again, is made up of 8 individual LEDs, as shown above. The LEDs
go in order of the alphabetical characters which you see. From first to last, the LEDs from
1-8 are a, b, c, d, e, f, g.
The decimal point would be the last pin.
To create an A, we would have to light LEDs, a,b,c,e,f,g. Thus to create it in code, it would
be represented by B11101110.
The full list of all the alphabetical characters with their corresponding code
we can display on the segment display is shown in the table below.
Alphabetical Character | Representation in Code |
A | B11101110 |
b | B00111110 |
C | B10011100 |
c | B00011010 |
d | B01111010 |
E | B10011110 |
F | B10001110 |
H | B01101110 |
h | B00101110 |
L | B00011100 |
l | B01100000 |
O | B11111100 |
o | B00111010 |
P | B11001110 |
S | B10110110 |
So now that you know how each numeral can be shown by deciding which LEDs to turn on,
let's see how we will wire the 7 segment LED display to the arduino.
Before we can do that, we must know the pinout of the 7
segment LED display. In our circuit, we will use a common cathode LED
display. This is a 7 segment LED display in which
all grounds of the LEDs are tied together.
The pinout of the common cathode LED display is shown below.
Common Cathode 7 Segment LED Display Pinout
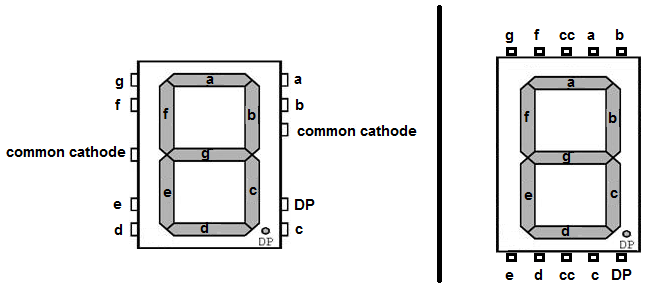
These are the 2 pinout diagrams of the common cathode 7
segment LED display. Some have the pins on the sides (laterally). Others
have the pins on the top and bottom. Thus,
we show both pinout diagrams.
You will need this to know how to hook up the display to the arduino board.
Note- Even though many different types of 7
segment LED displays follow the above schematics, it is not guaranteed.
The best way to know the connections is to obtain
the datasheet for the LED display in use or to buzz it out by connecting
power to each of the pins to see which lights up. If you don't get the
connections right, the circuit will not produce
the output of showing numerals 0-9. So it is very important to know
which pins are which.
Circuit Schematic
The schematic for the 7 segment LED display connected to the arduino is shown below.

The connections are fairly simple. We utilize 8 digital pins of the arduino and the ground terminal.
The written form of the above schematic is shown below for the pin connections.
7 Segment LED Display Pin | Connects to Arduino Digital Terminal ... |
a | 2 |
b | 3 |
c | 4 |
d | 6 |
e | 7 |
f | 9 |
g | 8 |
DP | 5 |
Note that the common cathode terminals connect to the ground terminal of the arduino.
Code
/*This is the code to show the alphabetical characters on a 7 segment LED display*/
//bits representing the alphabetical characters
const byte alphabet[16]= {
B11101110, //A
B00111110, //b
B10011100, //C
B00011010, //c
B01111010, //d
B10011110, //E
B10001110, //F
B01101110, //H
B00101110, //h
B00011100, //L
B01100000, //l
B11111100, //O
B00111010, //o
B11001110, //P
B10110110, //S
B00000000, //shows nothing
};
//pins for each segment (a-g) on the 7 segment LED display with the corresponding arduino connection
const int segmentPins[8]= { 5,8,9,7,6,4,3,2 };
void setup()
{
for (int i=0; i < 8; i++)
{
pinMode(segmentPins[i], OUTPUT);
}
}
void loop()
{
for (int i=0; i <=15; i++)
{
showDigit(i);
delay(1000);
}
delay(2000); //after LED segment shuts off, there is a 2-second delay
}
void showDigit (int number)
{
boolean isBitSet;
for (int segment=0; segment < 15; segment++)
{
isBitSet= bitRead(alphabet[number], segment);
digitalWrite(segmentPins[segment], isBitSet);
}
}
//bits representing the alphabetical characters
const byte alphabet[16]= {
B11101110, //A
B00111110, //b
B10011100, //C
B00011010, //c
B01111010, //d
B10011110, //E
B10001110, //F
B01101110, //H
B00101110, //h
B00011100, //L
B01100000, //l
B11111100, //O
B00111010, //o
B11001110, //P
B10110110, //S
B00000000, //shows nothing
};
//pins for each segment (a-g) on the 7 segment LED display with the corresponding arduino connection
const int segmentPins[8]= { 5,8,9,7,6,4,3,2 };
void setup()
{
for (int i=0; i < 8; i++)
{
pinMode(segmentPins[i], OUTPUT);
}
}
void loop()
{
for (int i=0; i <=15; i++)
{
showDigit(i);
delay(1000);
}
delay(2000); //after LED segment shuts off, there is a 2-second delay
}
void showDigit (int number)
{
boolean isBitSet;
for (int segment=0; segment < 15; segment++)
{
isBitSet= bitRead(alphabet[number], segment);
digitalWrite(segmentPins[segment], isBitSet);
}
}
The first block of code creates a byte named alphabet which store all the code needed to create the numbers 0-9 on the LED with a blank at the end.
The 11 characters are 0-9 and a blank.
The second block of code creates a constant integer called segmentPins which assigns the pins on the arduino that the 7 segment display is hooked up to.
The third block of code declares all pins on the arduino that the 7 segment LED display is hooked up to as output.
The fourth block of code calls a function called
showDigit() which is defined in the next block of code. This function
shows the alphabetical characters on the 7 segment LED
display. In between each numeral, there is a 1 second delay, as shown in
the line delay(1000). At the end of the loop when all characters have been shown, there is a 2-second delay
(delay(2000)).
The fifth block of code defines the function showDigit(). This displays the numbers defined in the constant byte alphabet.
Tuesday, April 1, 2014
Q:- What is L.E.D. ???
A light-emitting diode (LED) is a semiconductor device that emits visible light when an electric current passes through it. The light is not particularly bright, but in most LEDs it is monochromatic, occurring at a single wavelength.
The
output from an LED can range from red (at a wavelength of approximately
700 nanometers) to blue-violet (about 400 nanometers). Some LEDs emit
infrared (IR) energy (830
nanometers or longer); such a device is known as an infrared-emitting diode (IRED).
An LED or IRED consists of two elements of processed material called P-type
semiconductors and N-type semiconductors. These two elements are placed in direct contact, forming a region called the P-N junction. In this respect, the LED or IRED resembles most other diode
types, but there are important differences. The LED or IRED has a
transparent
package, allowing visible or IR energy to pass through. Also, the LED
or IRED has a large PN-junction area whose shape is tailored to the
application.
Benefits of LEDs and IREDs, compared with incandescent and fluorescent illuminating devices, include:
- Low power requirement: Most types can be operated with battery power supplies.
- High efficiency: Most of the power supplied to an LED or IRED is converted into radiation in the desired form, with minimal heat production.
- Long life: When properly installed, an LED or IRED can function for decades.
Typical applications include:
- Indicator lights: These can be two-state (i.e., on/off), bar-graph, or alphabetic-numeric readouts.
- LCD panel back-lighting: Specialized white LED's are used in flat-panel computer displays.
- Fiber optic data transmission: Ease of modulation allows wide communications bandwidth with minimal noise, resulting in high speed and accuracy.
- Remote control: Most home-entertainment "remotes" use IREDs to transmit data to the main unit.
- Optoisolator: Stages in an electronic system can be connected together without unwanted interaction.
Wednesday, March 26, 2014
LED ADVERTISING SCREENS
High Resolution LED Lamp and SMD Technology
When we started producing the first full colour LED modules . we saw a massive future potential for the technology within the advertising industry. Unfortunately back then full colour LED technology was extremely expensive and it was very difficult for media / marketing companies to see the benefits and recoup advertising revenue from the initial capital investment. This is the main reason why this technology became a popular rental solution and more suited to stadium applications and outdoor events.Today things are very different, the technology has advanced and component costs have reduced. The timing could not be better to launch a NEW range of modules specifically focused on advertising applications. This means smaller screen sizes with a higher resolution to meet customer’s advertising budgets. Our key focus have been to develop a number of high resolution modules using our high brightness SMD (3-in-1) technology and dot matrix boards. The result is a number of cost effective screens solutions for the signage, display and advertising industries.
Indoor / Window / Semi Outdoor Advertising Screens
Our new range of high resolution full color LED modules offers a costs effective solution to smaller scale digital billboard advertising, poster displays, banners and our LED light-box. Sai Display Board is focused on developing a number of screen solutions which differ from conventional LED displays by adding a whole new dimension to the use of the technology, creating movement and impact without the large price tag.
Digital LED Billboards (landscape or portrait)
The focus here is to offer smaller scale LED advertising boards using a high resolution module for closer viewing, making them ideal for all low level advertising applications within shopping centres, airports, bus/rail stations, visitor attractions, exhibition halls and outdoor pedestrian walkways. They also have a great impact for window advertising applications. This type of screen can be any size or shape (aspect) and also integrate well with larger static billboard poster displays.
Other LED advertising solutions include:
· LED 6 Sheet Poster System
· LED Light-box Sign
· LED Banner Screens
· LED TV
SMD (3-in-1) Technology
Sai Display Board has made many advances in its range of SMD (3-in-1) screen solutions over the last two years with the development of a new range of chips from Nichia and CREE. Utilising special techniques we have been able to increase brightness and contrast levels on the boards to create a costs effective range of full colour indoor / semi outdoor displays.
Indoor / Window / Semi-Outdoor Modular Screen Systems
Pixel Pitch - 6mm, 8mm, 10mm, 12mm
New for 2008 – 4mm pixel pitch.
Dot Matrix Technology
Utilising new manufacturing techniques our engineers have recently been able to develop a range of high brightness full colour dot matrix modules to create cost effective displays for the signage / advertising industry. These high resolution display modules are available in 6mm, 7.62mm and 10mm pixel pitch, with a brightness level of 2700 nits making them suitable for both indoor / window / semi outdoor applications. The board design means the display has a more even surface offering greater uniformity and colour blending across the surface.
Indoor / Window / Semi-Outdoor Modular Screen Systems
Pixel Pitch - 6mm, 7.62mm and 10mm
LED Lamp Technology
Our LED lamp technology is predominantly used for outdoor displays, but we have also recently developed a NEW type of economical flat LED lamp technology which we are currently utilising for our 7.62mm full color indoor screens.
Indoor Modular Screen Systems
Pixel Pitch - 7.62mm, 10mm and 12mm
12mm rental modules available with special cabinets.
Sai Display Board’s LED display solutions – utilize only the highest grade components available within the industry, to provide product quality and reliability. All our displays are produced under strict ISO standards and every component is checked and tested prior to delivery. We pride ourselves on having one of the most comprehensive warranties within the LED industry, offering 3 year warranty on all LED components we produce. Factory engineers are available to assist our dealers with any large installs, to commission the system and train your engineers on how to operate the control system / software and carry out general maintenance.
Standard Features
· Highest quality components (Japan / USA)
· Quality and reliability (ISO standards)
· Strict safety standards (CE / UL / ETL)
· 3 year comprehensive warranty
· Modular screen designs
· Factory installers (worldwide)
· Control system / software
· Spare parts / training / technical support
· OEM / custom designs
Recommended Applications
· Advertising / window displays
· Shopping centers / malls
· Visitor attractions / theme parks
· Indoor arenas / concert halls
· Exhibitions / conferences
· Airports / bus / rail stations
· LED video wall
Sai Display Board has been involved in the design of many custom display
solutions using LED technology. This has included the integration of LED
panels into the structure of buildings, creating artistic light
changing wall sculptures and numerous architectural lighting effects for
both corporate and commercial environments. Other applications have
included count down clocks to launch specific events and rotating LED
globes on the top of multi-story buildings.
By adopting a number of new technologies, Pro Display can create an array of exciting visual effects using LED's. The utilization of vibrant new colors such as true blue, green and white LED's has been a revelation – light years away from the old red tic-a-tape message boards.
Whether you are looking to create a simple color changing lighting effect for your building or an illuminated sign board, Sai Display Board have the solutions. Using our mirror vision technology we can create a number of different message board solutions that will simply blend in with your surroundings.
Recommended Applications
· Innovative display solutions
· Architectural lighting
· Retail / POS displays
· Mood / atmospheric lighting
· Lighting effects / entertainment
If you have a specific design, screen size and specification you require please send us with details and we will provide you with quote accordingly.
Sai Display Board’s LED display solutions – utilize only the highest grade components available within the industry, to provide product quality and reliability. All our displays are produced under strict ISO standards and every component is checked and tested prior to delivery. We pride ourselves on having one of the most comprehensive warranties within the LED industry. Factory engineers are available to assist our dealers with any large installs, to commission the system and train your engineers on how to operate the control system / software and carry out general maintenance.
By adopting a number of new technologies, Pro Display can create an array of exciting visual effects using LED's. The utilization of vibrant new colors such as true blue, green and white LED's has been a revelation – light years away from the old red tic-a-tape message boards.
Whether you are looking to create a simple color changing lighting effect for your building or an illuminated sign board, Sai Display Board have the solutions. Using our mirror vision technology we can create a number of different message board solutions that will simply blend in with your surroundings.
Recommended Applications
· Innovative display solutions
· Architectural lighting
· Retail / POS displays
· Mood / atmospheric lighting
· Lighting effects / entertainment
If you have a specific design, screen size and specification you require please send us with details and we will provide you with quote accordingly.
Sai Display Board’s LED display solutions – utilize only the highest grade components available within the industry, to provide product quality and reliability. All our displays are produced under strict ISO standards and every component is checked and tested prior to delivery. We pride ourselves on having one of the most comprehensive warranties within the LED industry. Factory engineers are available to assist our dealers with any large installs, to commission the system and train your engineers on how to operate the control system / software and carry out general maintenance.
OUTDOOR LED VIDEO SCREENS
Sai Display Board has been involved in the design and development of full colour LED displays since the technology evolved . Our engineers have vast experience in the industry having developed all kinds for LED solutions including message boards, graphic panels, time / temperature displays, scoreboard modules, full colour video screens and even LED light sculptures. Over the years we have installed thousands of LED displays into numerous industries – shops, shopping centres, corporate offices, factories, call centres, government buildings, transport links, stadiums and arenas.Outdoor LED Video Displays
Our new range of modular full colour LED screens offers a costs effective solution for big screen advertising and entertainment. We utilise a number of different LED technologies to bring you a choice of displays which should meet every budget. Pro Display recommends their LED lamp technology for most outdoor video screen applications.
LED Lamp Technology
Sai Display Board prides itself on its comprehensive range of full colour LED lamp solutions, utilising the very best lamp components from Japan / USA. Our LED lamp technology is mainly used for our range of outdoor LED screens as the brightness levels are high enough to compete with direct sunlight. Many of our full colour outdoor screens are created using ‘virtual pixel’ technology which has the effect of doubling the resolution for moving images. Depending on a customer’s requirements we can manufacture our LED lamp screens using two different types of lamp, which means we have an economy range and an exclusive range depending upon budget.
Outdoor Modular Screen Systems
Pixel Pitch - 10mm, 12mm, 16mm, 20mm, 25mm, 30mm and 40mm
12mm rental modules available with special cabinets.
SMD (3-in-1) Technology
Pro Display has made many advances in its range of SMD (3-in-1) screen solutions over the last two years with the development of a new range of chips from Nichia and CREE. Utilising special techniques we have been able to increase brightness and contrast levels on the boards to create a costs effective range of full colour displays.
Outdoor Modular Screen Systems
Pixel Pitch - 10mm and 16mm
The 16mm SMD board is utilised for our LED perimeter systems for football stadiums and sports arenas.
Semi-Outdoor Modular Screen Systems
Pixel Pitch - 6mm, 8mm, 10mm, 12mm
Sai Display Board’s LED display solutions utilize only the highest grade components available within the industry, to provide product quality and reliability. All our displays are produced under strict ISO standards and every component is checked and tested prior to delivery. We pride ourselves on having one of the most comprehensive warranties with the LED industry, offering 3 year warranty on all LED components we produce. Factory engineers are available to assist our dealers with any large installs, to commission the system and train your engineers on how to operate the control system / software and carry out general maintenance.
Applications
· Outdoor advertising / TV
· Shopping centers / malls
· Visitor attractions / theme parks
· Sports stadiums / arenas
· Outdoor events / concerts
· Airports / bus / rail stations
· LED video walls
Subscribe to:
Posts (Atom)